Traditional CI-CD vs gitops
Are you starting a new project, and stuck at making important decisions, and one big of these choices is how to deploy your code? In this article, we break down the differences between two popular approaches: the familiar Continuous Integration/Continuous Deployment (CI/CD) and the newer GitOps method. In your software delivery journey if you’re wondering which method to use for your project, stay with us as we explain these approaches in simple terms.
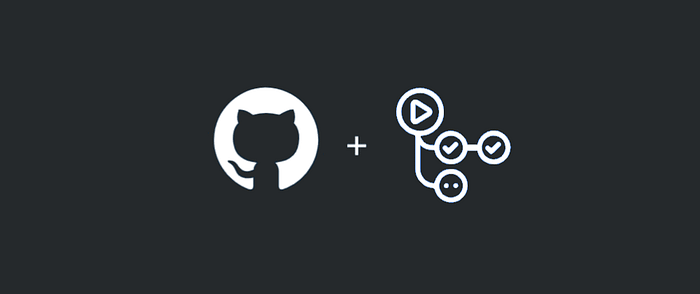
First, let’s understand how we’ve been implementing CI/CD traditionally,
Traditional CI/CD
Traditional CI/CD involves the automation of building, testing, and deploying applications through dedicated pipelines.
These pipelines are often triggered by events like code commits, and the focus is on the sequential execution of predefined steps.
Configuration and deployment artefacts are typically managed by CI/CD systems, and updates are pushed to target environments.
Code Example: Jenkins Pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build the application
sh 'mvn clean install'
}
}
stage('Test') {
steps {
// Run tests
sh 'mvn test'
}
}
stage('Deploy') {
steps {
// Deploy to the target environment
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
In the above Jenkinsfile, we witness a classic representation of a CI/CD pipeline. This script defines a series of procedural steps, commonly known as stages, responsible for building, testing, and deploying the application. Orchestrated by Jenkins, this CI/CD pipeline executes these stages sequentially, with explicit commands such as mvn clean install
for building and kubectl apply -f deployment.yaml
for deployment. The pipeline is designed to respond to predefined events, providing a controlled and systematic approach to software delivery.
now coming to gitops,
GitOps
GitOps, on the other hand, shifts the paradigm by using Git as the single source of truth for both application code and infrastructure. The desired state of the entire system, including application configurations and infrastructure definitions, is declared in a Git repository. Automation is achieved through continuous synchronization with this Git repository. Changes made in the repository automatically trigger updates in the target environment.
Instead of being event-driven like traditional CI/CD, GitOps continuously monitors the Git repository for changes and acts upon those changes. So basically, In a GitOps workflow, changes are submitted and detected by a GitOps agent that synchronizes them with the production environment.
Code Example: Argo CD for Kubernetes
# Application.yaml
apiVersion: argoproj.io/v1alpha1
kind: Application
metadata:
name: my-app
namespace: default
spec:
source:
repoURL: https://github.com/my-repo
path: my-app
destination:
server: 'https://kubernetes.default.svc'
namespace: my-namespace
In the above YAML file, we delve into the GitOps methodology, specifically suited for Argo CD. Unlike the procedural nature of traditional CI/CD, GitOps emphasizes a declarative approach. This configuration file declares the desired state of the application, outlining the source code’s location (repoURL
and path
) and the target Kubernetes cluster (server
and namespace
). Argo CD, in a continuous synchronization mode, monitors the specified Git repository (https://github.com/my-repo) for changes. Any modifications trigger an automatic adjustment of the actual state to align with the declared state. This Kubernetes-native GitOps configuration simplifies deployment, relying on a declarative Git repository as the single source of truth.
Let’s summarise. Key Differences:
1. Source of Truth:
— Traditional CI/CD relies on CI/CD systems for configuration and deployment.
— GitOps uses Git as the single source of truth for both code and infrastructure.
2. Declarative vs. Procedural:
— CI/CD often follows a procedural approach with predefined steps in pipelines.
— GitOps takes a declarative approach, where the desired state is declared in the Git repository, and the system automatically converges to this state.
3. Automation Trigger:
— In CI/CD, pipelines are typically triggered by events like code commits.
— GitOps systems continuously monitor the Git repository, automatically applying changes without the need for explicit triggers.
4. Immutable Infrastructure:
— GitOps often promotes the use of immutable infrastructure, where updates involve replacing the entire environment with a new version, enhancing reproducibility.
They can choose what works best for them, taking advantage of the strengths of each method or even combining them to build a smooth and effective way of working. It’s like having different tools in a toolbox — you pick the right one for the job at hand!